Table of Contents
1. Coding Tips
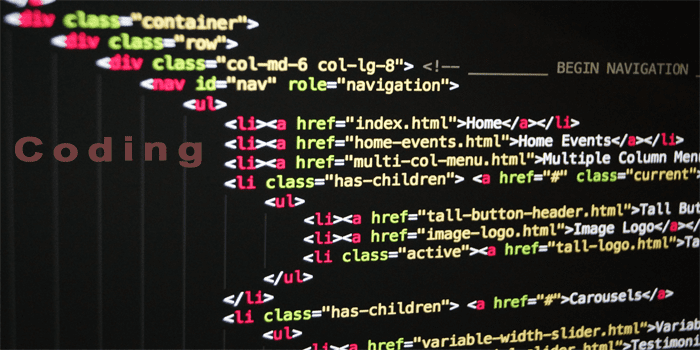
Coding tips are invaluable for improving your skills and efficiency as a programmer. Here are some essential tips to enhance your coding:
Use meaningful and descriptive variable names: Clear and concise variable names improve code readability, making it easier for you and others to understand the purpose of each variable.
Comment your code: Adding comments to your code helps explain complex logic or algorithms, making it easier for others (and yourself) to understand and maintain the code in the future.
Practice modular coding: Break down your code into smaller, reusable modules or functions. This not only promotes reusability but also improves code readability and maintainability. Keep your code DRY (Don’t Repeat Yourself): Avoid duplicating code by using functions, loops, and libraries whenever possible. This reduces the chances of introducing errors and makes code maintenance more manageable.
Follow coding style guidelines: Consistency in coding style improves readability and collaboration across a project. Adhere to established style guides or create your own, ensuring all team members follow the same conventions.
Test your code thoroughly: Writing unit tests and running them regularly helps catch bugs early on and ensures that your code functions correctly. Automated testing frameworks can streamline this process.
Stay updated with new technologies: The programming landscape is constantly evolving. Stay updated with the latest languages, frameworks, and tools to enhance your coding skills and efficiency.
Document your code: Documenting your code helps other developers understand its purpose, inputs, and outputs. Clear documentation saves time when revisiting code or collaborating with other team members.
Seek feedback and learn from experienced programmers: Embrace constructive criticism and continually seek feedback from more experienced programmers. Their insights and suggestions can help you improve your coding practices.
Don’t be afraid to refactor: Refactoring involves rewriting parts of your code to make it cleaner, more efficient, and easier to maintain. Regularly review your code for opportunities to refactor and optimize it.
2. Programming Tips
Programming tips offer guidance on strategies and techniques to become a more proficient programmer. Here are some essential programming tips:
Break down complex problems: When faced with a complex problem, break it down into smaller, manageable tasks. Solve each task independently before combining them to tackle the bigger problem.
Use libraries and frameworks: Utilize existing libraries and frameworks that provide pre-built functions and modules designed to simplify common programming tasks. This saves time and reduces the chances of reinventing the wheel.
Follow the SOLID principles: SOLID is an acronym for five design principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) that promote modular, maintainable, and extensible code.
Adopt a version control system: Version control systems like Git allow you to track changes to your code, collaborate with others, and easily revert back to previous versions if needed. Learn the basics of version control to streamline your programming workflow.
Learn different programming paradigms: Explore various programming paradigms such as procedural, object-oriented, functional, and declarative. Diversifying your programming knowledge enhances your problem-solving capabilities.
Practice clean code: Write code that is easy to read, understand, and follow. Utilize proper indentation, meaningful variable names, and consistent formatting. Clean code boosts readability and maintainability.
Embrace code reviews: Code reviews help uncover bugs, improve code quality, and facilitate knowledge sharing among team members. Actively participate in code reviews to enhance your programming skills.
Plan before coding: Invest time in planning your code structure and algorithms before diving into implementation. A well-thought-out plan saves time and minimizes the need for significant code refactoring later. Read code from experienced programmers: Read and analyze code written by experienced programmers to learn new techniques and gain insights into best practices. Platforms like GitHub offer a vast range of open-source projects to explore.
Stay curious and keep learning: The programming field is ever-evolving. Cultivate a thirst for knowledge and continuously learn new programming languages, frameworks, and techniques to stay ahead in your programming career.
3. Coding Tricks
Coding tricks refer to clever hacks or shortcuts that can streamline your coding process and improve productivity. Here are some useful coding tricks:
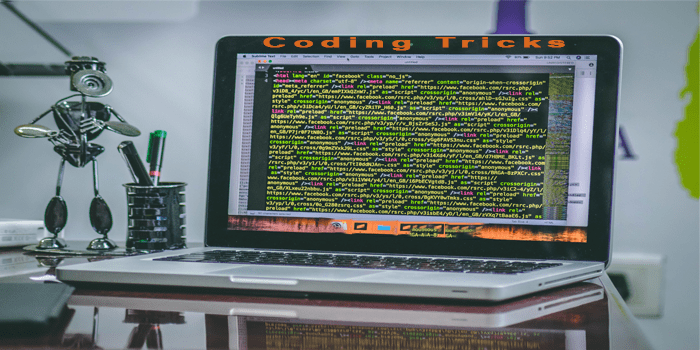
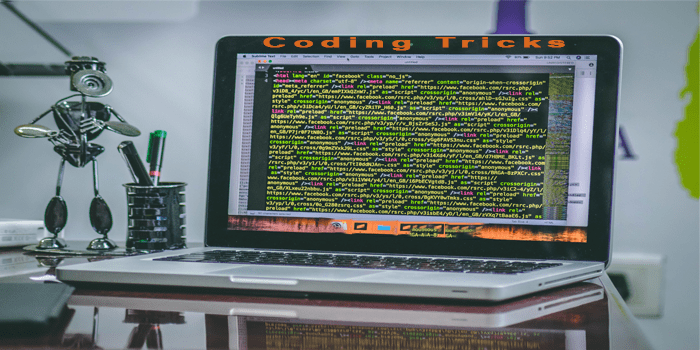
Utilize keyboard shortcuts: Familiarize yourself with keyboard shortcuts specific to your chosen text editor or IDE. These shortcuts can significantly speed up your coding workflow by reducing reliance on mouse clicks.
Leverage code snippets or templates: Many editors or IDEs offer the capability to create and utilize code snippets. Take advantage of this feature to quickly insert pre-written code segments for common tasks.
Master regular expressions: Regular expressions are powerful tools for pattern matching and manipulating text. Invest time in learning regular expressions, as they can simplify complex string operations.
Use code generation tools: When applicable, employ code generation tools that automatically generate repetitive or boilerplate code. This saves time and reduces the risk of introducing errors due to manual typing.
Employ code linting: Code linting tools analyze your code for potential errors, style inconsistencies, and best practice violations. Integrating these tools into your workflow improves code quality and adherence to coding standards.
Debug effectively: Learn debugging techniques and utilize debuggers provided by your programming environment. Debugging allows you to identify and fix issues more efficiently, speeding up the development process.
Profile your code: Profiling tools help identify performance bottlenecks in your code. By analyzing execution times and resource usage, you can optimize critical sections and improve overall efficiency.
Experiment with IDE plugins: Explore available plugins for your IDE, as these can enhance functionality and offer new features, such as code completion, syntax highlighting, and refactoring tools.
Utilize code revision history: Take advantage of code revision history tools, such as Git, to review changes made over time. This allows you to understand the evolution of your code and effectively manage versions.
Use online coding communities: Engage with online coding communities, such as Stack Overflow or GitHub, to learn from others, seek solutions to coding challenges, and contribute to open-source projects.
4. Programming Tricks
Programming tricks are smart techniques and methods that programmers can employ to streamline their development process. Here are some useful programming tricks:
Optimize algorithms and data structures: Choose algorithms and data structures tailored to the problem you’re solving. Optimization in this area can significantly improve program efficiency.
Employ memoization: Memoization is a technique where the results of expensive function calls are cached. This technique reduces redundant calculations and improves execution speed.
Understand time and space complexity: Familiarize yourself with time and space complexity analysis to assess the efficiency of your algorithms. Choose algorithms with lower time and space complexity for optimal performance.
Take advantage of parallel computing: When dealing with computationally intensive tasks, leverage parallel computing paradigms such as multi-threading or distributed computing to exploit the power of modern systems with multiple cores or nodes.
Use bitwise operations: Bitwise operations can be used for various optimizations and manipulations, especially when dealing with low-level programming or optimizing memory usage.
Explore compiler optimization flags: Compiler optimization flags allow you to maximize program performance by instructing the compiler to generate highly optimized machine code tailored to specific architectures.
Utilize caching: Implement caching mechanisms to store frequently accessed or expensive-to-compute data, reducing the need for repeated calculations.
Minimize I/O operations: Input/output operations can be costly in terms of time and resources. Minimize unnecessary I/O operations and batch operations where possible to optimize program performance.
Take advantage of lazy evaluation: Lazy evaluation defers the evaluation of an expression until its value is needed. This technique can improve performance by avoiding unnecessary computations.
Consider asynchronous programming: Asynchronous programming allows tasks to run concurrently without blocking the execution of other tasks. Utilize asynchronous programming paradigms and frameworks to maximize resource utilization and responsiveness.
5. Coding Techniques
Coding techniques encompass strategies and approaches that programmers can employ to write efficient and maintainable code. Here are some important coding techniques:
Object-oriented programming (OOP): OOP organizes code around objects or classes representing real-world entities. This technique improves code reusability, readability, and maintainability.
Functional programming (FP): FP treats computation as the evaluation of mathematical functions. It emphasizes immutability and avoids side effects, resulting in code that is easier to test, debug, and reason about.
Test-driven development (TDD): TDD involves writing tests before implementing functionality. This approach ensures the code meets requirements, promotes modular design, and facilitates refactoring.
Design patterns: Design patterns are proven solutions to recurring design problems in software development. Familiarize yourself with common design patterns such as Singleton, Factory, and Observer to improve code architecture and maintainability.
Model-View-Controller (MVC): MVC is an architectural pattern that separates the user interface (view), data (model), and business logic (controller) into distinct components. Applying MVC improves code organization and modularity.
Error handling: Implement robust error handling mechanisms to gracefully handle exceptions and avoid program crashes. Use try-catch blocks and appropriate exception handling techniques to detect and recover from errors.
Defensive programming: Write code that guards against unexpected inputs or errors. Validate user input, check for null or undefined values, and handle exceptional scenarios to create more robust software.
Event-driven programming: Event-driven programming responds to events or user actions by executing corresponding event handlers. This programming paradigm is commonly used in graphical user interfaces and game development.
Multi-threading and concurrency: Employ multi-threading techniques to perform tasks simultaneously, utilizing the capabilities of modern CPUs. Proper synchronization and thread management are necessary to avoid race conditions and deadlocks.
Aspect-oriented programming (AOP): AOP separates cross-cutting concerns (e.g., logging, error handling) from core business logic, enhancing code modularity and reusability.
6. Programming Techniques
Programming techniques encompass a variety of strategies and approaches used by programmers to solve problems efficiently. Here are some key programming techniques:
Divide and conquer: Divide complex problems into smaller, more manageable subproblems. Solve each subproblem independently and combine the results to obtain the final solution.
Dynamic programming: Dynamic programming breaks down a problem into overlapping subproblems and saves their solutions to avoid redundant calculations. This technique offers efficient solutions for problems with optimal substructure.
Greedy algorithms: Greedy algorithms make locally optimal choices at each step with the hope of finding a globally optimal solution. They are particularly useful for optimization problems, but may not always yield the best solution.
Backtracking: Backtracking is a trial-and-error approach used to find solutions by recursively exploring all possible choices. It is commonly employed in constraint satisfaction problems or combinatorial optimization.
Genetic algorithms: Inspired by the principles of natural selection, genetic algorithms iteratively evolve a population of potential solutions through selection, crossover, and mutation. These algorithms are useful for optimization problems without a clearly defined objective function.
Heuristics: Heuristics are problem-solving techniques that provide approximate solutions when an exact solution is not feasible in a reasonable time. They prioritize speed over accuracy and are commonly used in problem domains with large search spaces.
Branch and bound: Branch and bound solves combinatorial optimization problems by recursively partitioning the search space into smaller subspaces and bounding the search using upper and lower bounds.
Simulated annealing: Simulated annealing mimics the physical process of annealing in metals to find the optimal solution among a large set of potential solutions. It gradually explores the solution space while allowing some suboptimal moves to escape local optima.
Tabu search: Tabu search maintains a short-term memory of recently visited states or moves to escape local optima in the search space. It uses this memory to guide the search towards potentially better solutions.
Linear programming: Linear programming solves optimization problems with linear objective functions and constraints. It determines the best possible outcome by finding an optimal combination of decision variables that satisfy the constraints.
7. Coding Advice
Coding advice encompasses general recommendations and insights to help programmers write better code. Here are some valuable coding advice tips:
Read and respect existing code conventions: Projects often have established coding conventions. Familiarize yourself with these conventions and follow them consistently to ensure code consistency and collaboration.
Write code for readability: Strive for code that is easy to understand and maintain. Use meaningful variable names, avoid overly complex expressions, and add comments where necessary. Prioritize readability over clever or cryptic code.
Keep functions and methods concise: Aim for smaller functions or methods that perform a specific task. This improves code modularity, reusability, and testability.
Don’t optimize prematurely: Avoid premature optimization, as it can lead to overcomplicated and less maintainable code. Focus on writing clear and correct code first, then optimize performance bottlenecks when necessary.
Embrace single responsibility principle: Each function, class, or module should have a single, well-defined responsibility. This increases code maintainability and makes it easier to identify and fix bugs.
Use version control effectively: Commit your code frequently and log meaningful commit messages. Utilize branching strategies and merge workflows suitable for your project to ensure efficient collaboration and version management.
Perform code reviews: Engage in code reviews to receive feedback from peers. Code reviews help identify issues, improve code quality, and foster a culture of knowledge sharing within the development team.
Keep up with documentation: Document your code, APIs, and libraries to make them self-explanatory. Well-documented code saves time for future developers who may need to understand or modify your code.
Validate user input religiously: Ensure that user input is thoroughly validated and sanitized to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS) attacks.
Continuously learn and improve: The programming landscape evolves rapidly, so embrace lifelong learning. Regularly update your skills through courses, books, conferences, and online resources to stay up-to-date with best practices and emerging technologies.
8. Programming Advice
Programming advice offers guidance and recommendations to programmers for improving their development process. Here are some important programming advice tips:
Understand the problem domain: Gain a deep understanding of the problem you’re solving before starting the implementation. This helps in designing appropriate data structures, algorithms, and logic.
Break down tasks and set achievable goals: Divide larger tasks into smaller, manageable subtasks. Setting achievable goals promotes a sense of accomplishment and progress throughout the development process.
Consider edge cases: Take into account edge cases and boundary conditions during implementation and testing. Robust software handles unexpected scenarios gracefully, ensuring error-free execution.
Write self-documenting code: Strive to write code that is self-explanatory by using descriptive function and variable names. This reduces the reliance on comments and minimizes confusion when revisiting code later.
Prioritize code readability over performance: While performance is crucial, readable code should be a priority. Only optimize for performance when performance benchmarks prove it necessary.
Use meaningful and consistent naming conventions: Adopt meaningful and consistent naming conventions for variables, functions, and classes. Naming should reflect the purpose and context of each entity while adhering to industry standards.
Minimize code duplication: Repeated code introduces maintenance overhead and increases the likelihood of introducing bugs. Extract repetitive code into functions, classes, or modules to promote code reuse and maintainability.
Plan for change: Anticipate future modifications and design your code accordingly. Write loosely coupled code that allows for easier enhancements or additions in the future.
Debug with a systematic approach: When encountering bugs or issues, approach debugging systematically by isolating the problem, gathering relevant information, and testing potential solutions incrementally.
Foster open communication: Foster open communication and collaboration within development teams. Encourage constructive feedback, knowledge sharing, and cross-team cooperation to improve code quality and productivity.
9. Coding Best Practices
Coding best practices encompass a set of guidelines and recommendations that developers should follow to produce high-quality code. Here are some essential coding best practices:
Follow modular programming: Divide your code into small, well-defined modules or functions. Each module should encapsulate a specific task or functionality, promoting reusability and enhancing maintainability.
Write readable and descriptive code: Use descriptive variable and function names that convey their purpose. Maintain consistent code formatting, indentation, and naming conventions to improve readability.
Avoid magic numbers and hard-coded values: Replace hard-coded values in your code with constants or configuration variables. This improves code maintainability and makes it easier to modify values when needed.
Use meaningful comments: Add comments to clarify complex logic, explain the purpose of functions or classes, and document any non-obvious decision-making within your code.
Keep functions and methods short and focused: Limit the length of your functions and methods to improve code readability and make them easier to understand. Avoid bloated, overly complex functions that are difficult to comprehend.
DRY (Don’t Repeat Yourself) principle: Avoid duplicating code by promoting code reuse. Extract commonly used functionality into functions, classes, or libraries to centralize logic and reduce code repetition.
Handle errors gracefully: Implement robust error handling by utilizing appropriate exception handling mechanisms. Avoid suppressing or ignoring errors, providing meaningful error messages for debugging purposes.
Write testable code: Design code that is easily testable by unit tests. Consider dependencies, separation of concerns, and modular design principles while writing code to facilitate testing.
Perform regular code refactoring: Refactor your code periodically to improve its structure, readability, and performance. This includes simplifying complex logic, removing dead code, and ensuring adherence to coding best practices.
Conduct code reviews: Engage in regular code reviews with peers to gather feedback, identify potential issues, and ensure code quality. Code reviews help catch bugs, promote knowledge sharing, and align with coding
10. Better programmer
Plan before you start coding: Take the time to understand the problem you’re trying to solve and break it down into smaller, manageable tasks. This will help you organize your thoughts and prevent unnecessary mistakes. Write clean and readable code: Use proper indentation, meaningful variable and function names, and consistent formatting. This will make your code easier to understand and maintain. Comment your code: Add comments to explain your thought process, document important decisions, or provide clarification for complex sections of code. This will make it easier for you and others to understand and modify your code in the future.
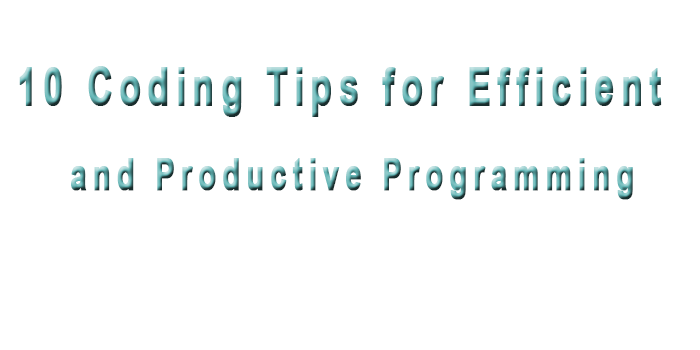
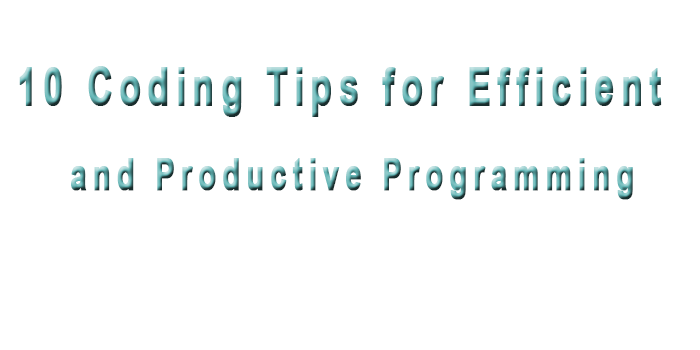
Powered by Bestwritebot.com , Otips , Free Video Downloader , softwarestore , free online game hub